GraphQL is an open-source data query and manipulation language for APIs, and a runtime for fulfilling queries with existing data. It has become increasingly popular since Facebook released it in 2015. GraphQL is similar to REST in that it is an API used to retrieve data, but there are some key differences. You can add GraphQL to any WordPress site by using the WPGraphQL plugin. Go ahead and install it on your site if you want to try out the examples in this post.
GraphQL Basics
GraphQL provides a single endpoint to query against which is typically /graphql. Queries are sent to the endpoint via POST requests. Here’s a simple query that pulls back all the posts in a WordPress instance:
query {
posts {
nodes {
id
title
slug
}
}
}
The result will be a JSON object that looks something like this:
{
"data": {
"posts": {
"nodes": [
{
"id": "cG9zdDozOQ==",
"title": "Another Post",
"slug": "another-post"
},
{
"id": "cG9zdDox",
"title": "Hello World!",
"slug": "hello-world"
}
]
}
}
}
Code language: JSON / JSON with Comments (json)
A key tenet of GraphQL is that it only returns the data you request. This is particularly important when we’re retrieving data on a mobile device or over a slow connection. The less data we need to transfer, the faster the result!
Testing Queries In GraphiQL
GraphQL provides an IDE for testing queries and exploring the schema called GraphiQL which WPGraphQL makes available on our sites. Once you install the plugin, you’ll see the WPGraphiQL IDE logo in the top menu bar of the WP Admin interface.
Let’s explore the interface for GraphiQL. Here’s what the overall interface looks like when you click the link in the WP Admin menu bar to navigate to it:
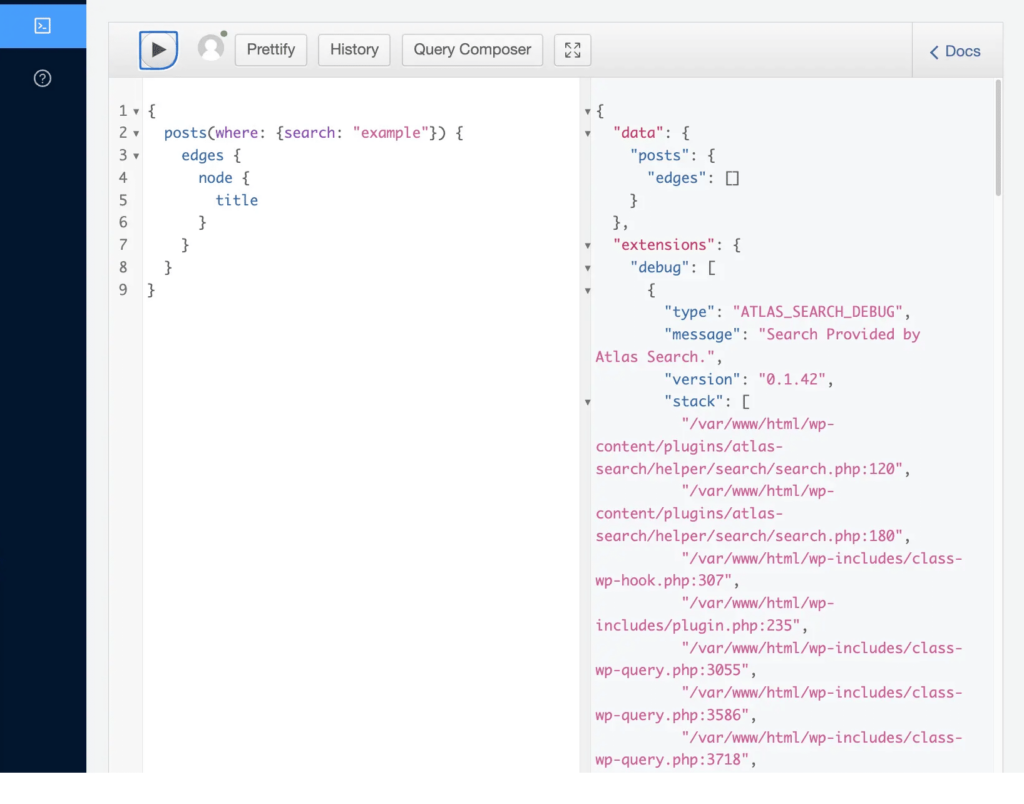
On the left, you’ll see a tree navigation that allows you to explore the schema that is exposed via WPGraphQL. You should see some familiar WordPress types like posts, pages, and comments. The middle section of the IDE is where you can write a query to run against the GraphQL server, and the right side of the interface displays the results of your query.
The GraphQL schema allows for relationships between data types. For instance, a WordPress post has an author. There’s a relationship between posts and users called author. Here’s a query that pulls back posts with their author:
query {
posts {
nodes {
id
title
slug
author {
node {
id
name
}
}
}
}
}
The result should looks like this:
{
"data": {
"posts": {
"nodes": [
{
"id": "cG9zdDox",
"title": "Hello World!",
"slug": "hello-world",
"author": {
"node": {
"id": "dXNlcjoy",
"name": "Matt Landers"
}
}
}
]
}
}
}
Code language: JSON / JSON with Comments (json)
GraphQL Relay Specification
At this point, you may notice something a bit odd in the queries and results. There’s an extra layer of nesting in node(s). The reason for the extra layers is that WPGraphQL follows the Relay Specification for GraphQL. The documentation explains the reason for implementing this specification here. In short, you typically don’t want to return every item for a type (i.e. post). Imagine a site with hundreds or thousands of blog posts. We don’t want to return all that data every time. We probably want to allow the user to page through the posts or infinitely scroll. The Relay Spec provides a standard way to get page information for any “node” in the schema.
Pagination In WPGraphQL
Let’s explore how we can paginate our results to limit the number of posts we return. Here’s an initial query to grab a page of posts with 2 entries. Notice that we pass an argument to posts that says we only want the first 2 posts.
{
posts(first: 2) {
pageInfo {
hasNextPage
endCursor
}
nodes {
id
title
slug
}
}
}
Now, let’s look at the result and see how we can grab the next page:
{
"data": {
"posts": {
"pageInfo": {
"hasNextPage": true,
"endCursor": "YXJyYXljb25uZWN0aW9uOjM4"
},
"nodes": [
{
"id": "cG9zdDozOQ==",
"title": "Another Nother Post",
"slug": "another-nother-post"
},
{
"id": "cG9zdDozOA==",
"title": "Another Post",
"slug": "another-post"
}
]
}
}
}
Code language: JSON / JSON with Comments (json)
In our query, we requested the pageInfo
. We asked if there’s another page of posts and what the ending cursor is for the current page of posts. Now, we can get the next page of posts by using the ending cursor. Notice that we pass 2 parameters to posts this time saying that we want the first 2 posts after the ending cursor that was provided from the previous query:
{
posts(first: 2, after: "YXJyYXljb25uZWN0aW9uOjM4") {
pageInfo {
hasNextPage
endCursor
}
nodes {
id
title
slug
}
}
}
Code language: JavaScript (javascript)
The query above is going to ask for 2 more posts and indicate that the posts should be after the previous endCursor
. We end up with a response similar to the following:
{
"data": {
"posts": {
"pageInfo": {
"hasNextPage": false,
"endCursor": "YXJyYXljb25uZWN0aW9uOjQy"
},
"nodes": [
{
"id": "cG9zdDo0Mg==",
"title": "The last post",
"slug": "the-last-post"
},
{
"id": "cG9zdDo0MQ==",
"title": "Some other Post",
"slug": "some-other-post"
}
]
}
}
}
Code language: JSON / JSON with Comments (json)
Notice the result above indicates "hasNextPage": false
. This is how WPGraphQL lets us know there are no more posts to fetch.
GraphQL Versus REST
If you haven’t already, read our post on the WordPress REST API. That post will walk you through a similar path as this posts only with the REST API. At the end of the day the choice is yours whether to use REST or GraphQL. WPGraphQL, and more broadly GraphQL, is gaining popularity because of its easy integration with frontend clients. There are certainly pros and cons to both, which warrants a separate post! Whatever you decide, hopefully this post helped familiarize you with GraphQL and the WPGraphQL plugin for WordPress.