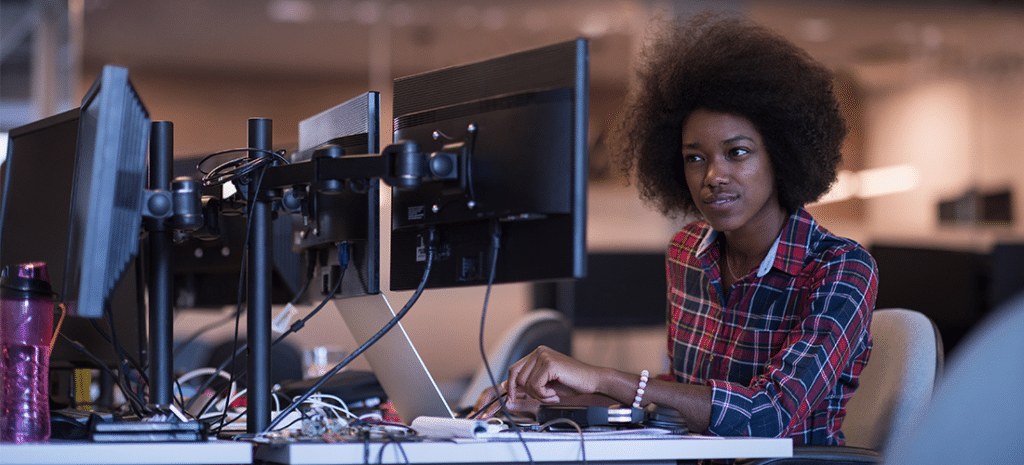
How to Animate with CSS
We’ve gotten pretty used to seeing animations on websites and enjoying the energy and variety they bring to web design. They’re eye catching, a great way to add some visual interest to a site, and generally make the experience more exciting for your users.
While traditionally achieved with GIFs, SVGs, WebGL, and background videos, animations can also be efficiently created with CSS. Browser support for CSS animations has greatly improved and is becoming quite popular — compatible browsers include Firefox 5+, IE 10+, Chrome, Safari 4+, and Opera 12+.
Today I’ll walk you through the basics of creating a CSS animation with a step-by-step demo. Stick around after that and check out five examples of animations. You’ll be able to use all these ideas to create animations for your own projects!
CSS Animation Basics
Obviously, it’s important to cover the basics of how animations work before we dive into the five fun CSS animations.
For example, keyframes are a key component to CSS animations. You may be familiar with that term if you’ve worked with Adobe Flash or have experience with video editing. In that case, the term keyframe is just what you would think: it’s a way to specify a certain action.
You may have come across @keyframes
in a CSS stylesheet before. Inside this @keyframes
is where you define the styles and stages for the animation. Here’s a great example of a fadeout effect:
@-webkit-keyframes fadeOut {
0% {
opacity: 1;
}
100% {
opacity: 0;
}
The basics of the fadeout keyframe we just created include:
- A descriptive name: in this case, fadeOut.
- Stages of the animation: From was set to 0% and To was set to 100%.
- CSS styles that will be applied at each stage.
By default, the From will be at 0% and the To will be at 100%, since no other stages are specified in this example.
Specific Actions with Animation Subproperties
We need to do a little more to style things — we need to style the animation
property with subproperties. If keyframes define what the animation will look like, then animation sub-properties define specific rules for the animation. These let you configure the timing, duration, and other key details of how the animation sequence should progress.
The animation property is used to call @keyframes
inside a CSS selector. Animations can and will often have more than one subproperty. Here are examples of subproperties:
- Animation-name: name of the
@keyframes
at-rule, which describes the animation’s keyframes. The namefadeOut
in the previous example is an example of animation-name. - Animation-duration: length of time that an animation should take to complete one full cycle.
- Animation-timing-function: timing of the animation, specifically how the animation transitions through keyframes. This function has the ability to establish acceleration curves. Examples are linear, ease, ease-in, ease-out, ease-in-out, or cubic-bezier.
- Animation-delay: delay between the time the element is loaded and the beginning of the animation.
- Animation-iteration-count: number of times the animation should repeat. Want the animation to go on forever? You can specify infinite to repeat the animation indefinitely.
- Animation-direction: whether or not the animation should alternate direction on each run through the sequence or reset to the start point and repeat itself.
- Animation-fill-mode: values that are applied by the animation both before and after has executed.
- Animation-play-state: with this option, you are able to pause and resume the animation sequence. Examples are none, forwards, backwards, or both.
Putting it All Together for Best Browser Support
There’s a lot going on here, and the terminology can be a bit confusing. But we now know that keyframes define what the animation will look like as well as different animation stages, and that the animation property uses subproperties to define animation options like delay, direction, timing, and so on.
You are probably familiar with vendor or browser prefixes — those are necessary when working with animations. We need to make sure that we have the best browser support. Here are the standard browser prefixes:
- Chrome & Safari:
-webkit-
- Firefox:
-moz-
- Internet Explorer:
-ms-
Internet Explorer 10 does not require a prefix for transitions, but all transforms require the prefix. Opera is covered because it recognises WebKit styles.
Transition start
-webkit-transition
-moz-transition
transition
Transform start:
-webkit-transform
-moz-transform
-ms-transform
transform
Five animations in action
Now that we’ve covered the basics, let’s create some code to put to use!
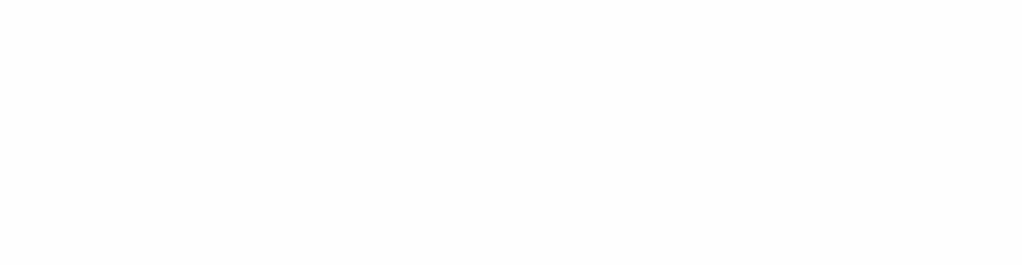
Animation One: circle to square
Let’s go detailed on this first one, so we can make sure we understand all the concepts we’ve covered so far. The animation will start out as a circle and morph into a square.
Creating a div to start with is a great way to test out the animation:
<div class=”animationOne”>
</div>
Now let’s get our @keyframes
set up. This animation will have five stages because a square has four sides and we need to have a 0% starting point. Use prefixes, as shown below, but for the rest of the tutorial we’ll keep it simple with just the basics.
@-webkit-keyframes circle-to-square {
0%{}
25%{}
50%{}
75%{}
100%{}
}
@-moz-keyframes circle-to-square {
0%{}
25%{}
50%{}
75%{}
100%{}
}
@-ms-keyframes circle-to-square {
0%{}
25%{}
50%{}
75%{}
100%{}
}
@keyframes circle-to-square {
0%{}
25%{}
50%{}
75%{}
100%{}
}
Now, let’s create some styles to determine what the border radius will appear to be at each stage:
@keyframes circle-to-square {
0% {
border-radius:50%;
}
25% {
border-radius:50% 50% 50% 0;
}
50% {
border-radius:50% 50% 0 0;
}
75% {
border-radius:50% 0 0 0;
}
100% {
border-radius:0 0 0 0;
}
}
Now add the background-color
property to help differentiate each stage of the animation:
@keyframes circle-to-square {
0% {
border-radius:50%;
background-color: #6a9bea;
}
25% {
border-radius:50% 50% 50% 0;
background-color: #90b3ec;
}
50% {
border-radius:50% 50% 0 0;
background-color: #b0c7ec;
}
75% {
border-radius:50% 0 0 0;
background-color: #cad7ec;
}
100% {
border-radius:0 0 0 0;
background-color: #dfe3e9;
}
}
Finally, let’s apply the animation to the test div:
.animationOne {
width: 200px;
height: 200px;
-webkit-animation: circle-to-square 2s 1s infinite alternate;
-moz-animation: circle-to-square 2s 1s infinite alternate;
-ms-animation: circle-to-square 2s 1s infinite alternate;
animation: circle-to-square 2s 1s infinite alternate;
}
The animation property is typically written in shorthand, so here’s what’s actually going on in the code:
- The animation-name is
circle-to-square
. - The animation-duration is
2s
. - The animation-delay is
1s
. - The animation-iteration-count is
infinite
, so it will carry on indefinitely. - And the animation-direction is
alternate
. This means it will play from beginning to end and go back to the beginning.
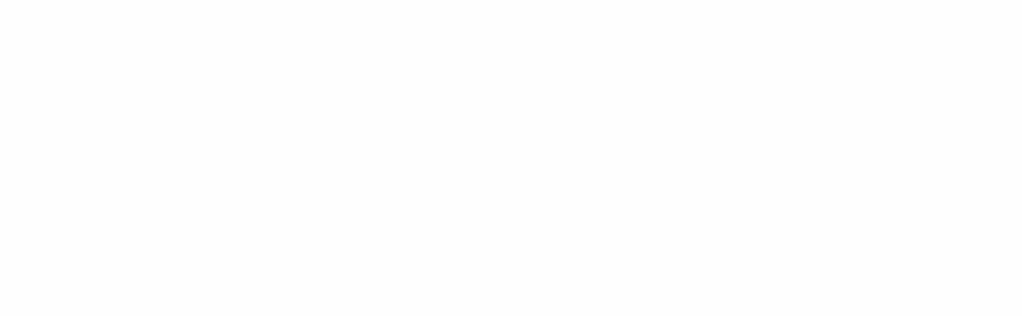
Animation Two: rotation
This animation allows for the rotation of an object.
@keyframes full-rotate {
0% {
transform: rotate(0deg);
}
25% {
transform: rotate(45deg);
}
50% {
transform: rotate(90deg);
}
75% {
transform: rotate(135deg);
}
100% {
transform: rotate(180deg);
}
}
To keep our animation projects separate, remember to create another div for this second example. I added a background color because we’ll be adding the animation to the div itself for this example.
The duration for this animation is two seconds, with a three second delay. The animation-iteration-count is five, so this project will animate five times before stopping. The animation plays in reverse every odd time (1,3,5,… ) and in a normal direction every even time (2,4,6,… ).
.animationTwo {
width: 200px;
height: 200px;
background-color: #ccc;
animation: full-rotate 2s 3s 5 alternate-reverse;
}

Animation Three: expand and flicker
This is a great animation for a button because it’s more eye catching and prominent in comparison to other elements on the page. This might also be something worthwhile to include on a :hover
state.
@keyframes button-flicker {
0% {
transform: scale(1);
}
30% {
transform: scale(1);
}
40% {
transform: scale(1.08);
}
50% {
transform: scale(1);
}
60% {
transform: scale(1);
}
70% {
transform: scale(1.05);
}
80% {
transform: scale(1);
}
100% {
transform: scale(1);
}
}
.btn.pulse {
background: #3498db;
background-image: -webkit-linear-gradient(top, #3498db, #2980b9);
background-image: -moz-linear-gradient(top, #3498db, #2980b9);
background-image: -ms-linear-gradient(top, #3498db, #2980b9);
background-image: -o-linear-gradient(top, #3498db, #2980b9);
background-image: linear-gradient(to bottom, #3498db, #2980b9);
-webkit-border-radius: 28;
-moz-border-radius: 28;
border-radius: 28px;
font-family: Arial;
color: #ffffff;
font-size: 20px;
padding: 10px 20px 10px 20px;
text-decoration: none;
animation: button-flicker 5000ms infinite linear;
}
To use test this, we need the following starter div:
.animationThree {
width: 200px;
height: 200px;
}
<div class=”animationThree>
<a class="btn pulse" href="">Click me</a>
</div>

Animation Four: text slide
Let’s add some animation to text. This project makes your text slide in once from the left.
@keyframes slide-text {
from {
margin-left: 100%;
width: 200%;
}
to {
margin-left: 0%;
width: 100%;
}
}
h1.slide {
animation-name: slide-text;
animation-duration: 3s;
}

Animation Five: fade in
Do you have some surprise content? Check out this fade in animation for content that you want to appear at a later moment. You’ve got a lot of options here: The fade in can be fast or slow, happen once or multiple times, and so on.
@keyframes fade {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.animationFive {
width: 200px;
height: 200px;
}
.animationFive img{
animation-name: fade;
animation-duration: 3s;
}
<div class=”animationFive”>
<img src=".." />
</div>
CSS3 animations are fantastic for getting your website moving and grooving! Now that you have the basic concepts down for creating animations, the animation possibilities are endless. What will you animate?